Windows Forms Application
In this tutorial, you will configure a C# or VB.NET project for a Windows Forms application and use ImageGear .NET PDF capabilities. You will also learn how to open a PDF or PS file, display it on the screen, and Save the document as a new file.
For local development, ImageGear .NET must be installed from the product installer. This will ensure that licensing is set up properly for local development. Once ImageGear .NET is installed, projects can use NuGet for building and deployment.
The following tutorial refers specifically to 64-bit installations; for 32-bit installations:
- Your project should already be set to compile to target Debug and x86, and you should have the directory: $YOURLOCALPROJ\bin\x86\Debug\.
- Throughout these instructions, replace x64 with x86.
- The 32-bit ImageGear binaries are found in $INSTALLDIR\ImageGear .NET v24\Bin\
Using the desired version of Visual Studio (2010 or later):
- Create a new "Windows Forms Application" project, using C# or VB.NET, and name the project: my_first_PDF_WINFORMS_project
- If you installed ImageGear .NET 64-bit, using the Configuration Manager, create a new project platform (if you don’t have one already) for x64. Make sure your project is set to compile targeting Debug and x64. Make sure you now have $YOURLOCALPROJ\bin\x64\Debug\, and if is not there, create it.
- Add references and required resources into your projects in one of the following ways:
- Recommended: use our NuGet Packages. For this project, you need the following packages:
- Manually:
- Copy all files (and folders) inside $INSTALLDIR\ImageGear .NET v24 64-bit\Bin\ to your local output bin directory in your project (i.e., $YOURLOCALPROJ\bin\x64\Debug\ ).
- Add the following references to your project from $YOURLOCALPROJ\bin\x64\Debug\:
- ImageGear24.Core.dll
- ImageGear24.Evaluation.dll
- ImageGear24.Formats.Common.dll
- ImageGear24.Formats.Pdf.dll
- ImageGear24.Presentation.dll
- ImageGear24.Formats.Vector.dll
- ImageGear24.Windows.Forms.dll
Your output target directory should be set to $YOURLOCALPROJ\bin\x64\Debug\ .
- Next, add the ImageGear Windows Forms tools to use in your GUI:
- In Visual Studio, go to Tools > Choose Toolbox Items...
- In the .NET Framework Components tab, click Browse and navigate to $INSTALLDIR\ImageGear .NET v24\Bin\, select ImageGear24.Windows.Forms.dll, and click Open.
- You will see the ImGearMagnifier, ImGearPageView, ImGearPan, and ImGearThumbnailCtl controls added to the list of components. Make sure they are checked and click OK.
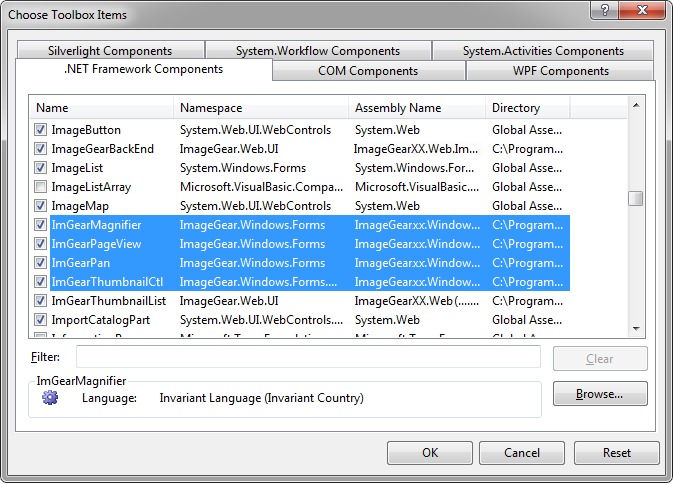
Next, you will add some pertinent controls to your form. For this tutorial, keep the default names for the controls.
- First, create the menu on the form. From the Windows Forms toolbox, drag a MenuStrip control onto the form. Create a menu called File. Under File, add Load, Save, Next Page, and Previous Page.
- Second, also from the toolbox, drag the ImGearPageView control onto the form. This control will be used to display the PDF document. Set the Dock (in the Layout group) property of the imGearPageView1 control to Fill. This will cause the control to resize as the form resizes.
- For the last control, add a statusStrip control. After it has been added, right-click on the control and insert a StatusLabel into it. This control will be used to keep track of the displayed page number.
- Double-click each added menu item to create a handler.
- At this point your project is ready for some code. The following code can be used to load a PDF or PS file, view it using an ImGearPageView control, and save it as new file. In the next step, we will go over some important areas of this sample code.
C# |
Copy Code |
using System;
using System.IO;
using System.Windows.Forms;
using ImageGear.Core;
using ImageGear.Display;
using ImageGear.Formats;
using ImageGear.Formats.PDF;
using ImageGear.Evaluation;
namespace my_first_PDF_WINFORMS_project
{
public partial class Form1 : Form
{
// Create empty IG document object.
private ImGearDocument igDocument = null;
private int currentPageIndex = 0;
public Form1()
{
// Initialize evaluation license.
ImGearEvaluationManager.Initialize();
ImGearEvaluationManager.Mode = ImGearEvaluationMode.Watermark;
// Add support for PDF and PS files.
ImGearCommonFormats.Initialize();
ImGearFileFormats.Filters.Insert(0, ImGearPDF.CreatePDFFormat());
ImGearFileFormats.Filters.Insert(0, ImGearPDF.CreatePSFormat());
ImGearPDF.Initialize();
InitializeComponent();
}
private void loadToolStripMenuItem_Click(object sender, EventArgs e)
{
OpenFileDialog openFileDialogLoad = new OpenFileDialog();
// Allow only PDF and PS files.
openFileDialogLoad.Filter =
ImGearFileFormats.GetSavingFilter(ImGearSavingFormats.PDF) + "|" +
ImGearFileFormats.GetSavingFilter(ImGearSavingFormats.PS);
if (DialogResult.OK == openFileDialogLoad.ShowDialog())
{
using (FileStream inputStream =
new FileStream(openFileDialogLoad.FileName, FileMode.Open, FileAccess.Read, FileShare.Read))
{
try
{
// Load the entire the document.
igDocument = ImGearFileFormats.LoadDocument(inputStream);
}
catch (ImGearException ex)
{
// Perform error handling.
MessageBox.Show(ex.Message);
}
}
// Render first page.
renderPage(0);
}
}
private void saveToolStripMenuItem_Click(object sender, EventArgs e)
{
// Check if document exists.
if (igDocument == null)
return;
string filename = "";
// Open File dialog. For this sample, just allow PDF or PS.
ImGearSavingFormats savingFormat = ImGearSavingFormats.PDF;
using (SaveFileDialog fileDialogSave = new SaveFileDialog())
{
fileDialogSave.Filter = ImGearFileFormats.GetSavingFilter(ImGearSavingFormats.PDF) + "|" +
ImGearFileFormats.GetSavingFilter(ImGearSavingFormats.PS);
fileDialogSave.OverwritePrompt = true;
if (fileDialogSave.ShowDialog(this) != DialogResult.OK)
{
return;
}
filename = fileDialogSave.FileName;
}
// Save to output file.
using (FileStream outputStream = new FileStream(filename, FileMode.Create, FileAccess.ReadWrite))
{
try
{
// Save the page in the requested format.
ImGearFileFormats.SaveDocument(
igDocument,
outputStream,
0,
ImGearSavingModes.OVERWRITE,
savingFormat,
null);
MessageBox.Show("Saved {0}", filename);
}
// Perform error handling.
catch (Exception ex)
{
MessageBox.Show(string.Format("Could not save file {0}. {1}", filename, ex.Message));
return;
}
}
}
private void renderPage(int pageNumber)
{
// Create page to hold the content.
ImGearPage igPage = null;
// Load a single page from the loaded document.
try
{
igPage = igDocument.Pages[pageNumber];
if (igPage != null)
{
// Create a new page display to prepare the page for being displayed.
ImGearPageDisplay igPageDisplay = new ImGearPageDisplay(igPage);
// Associate the page display with the page view.
imGearPageView1.Display = igPageDisplay;
// Cause the page view to repaint.
imGearPageView1.Invalidate();
currentPageIndex = pageNumber;
toolStripStatusLabel1.Text = string.Format("{0} of {1}", pageNumber + 1, igDocument.Pages.Count);
}
}
catch (ImGearException ex)
{
// Perform error handling.
MessageBox.Show(ex.Message);
}
}
// Properly dispose of ImGearPDF and other objects.
protected override void OnFormClosed(FormClosedEventArgs e)
{
ImGearPDF.Terminate();
imGearPageView1.Display = null;
}
private void nextPageToolStripMenuItem_Click(object sender, EventArgs e)
{
if (igDocument != null)
if (currentPageIndex < igDocument.Pages.Count-1)
renderPage(currentPageIndex + 1);
}
private void previousPageToolStripMenuItem_Click(object sender, EventArgs e)
{
if (igDocument != null)
if (currentPageIndex > 0)
renderPage(currentPageIndex - 1);
}
}
} |
VB.NET |
Copy Code |
Imports System.IO
Imports System.Windows.Forms
Imports ImageGear.Core
Imports ImageGear.Display
Imports ImageGear.Formats
Imports ImageGear.Formats.PDF
Imports ImageGear.Evaluation
Namespace my_first_PDF_WINFORMS_project
Public Partial Class Form1
Inherits Form
' Create empty IG document object.
Private igDocument As ImGearDocument = Nothing
Private currentPageIndex As Integer = 0
Public Sub New()
' Initialize evaluation license.
ImGearEvaluationManager.Initialize()
ImGearEvaluationManager.Mode = ImGearEvaluationMode.Watermark
' Add support for PDF and PS files.
ImGearCommonFormats.Initialize()
ImGearFileFormats.Filters.Insert(0, ImGearPDF.CreatePDFFormat())
ImGearFileFormats.Filters.Insert(0, ImGearPDF.CreatePSFormat())
ImGearPDF.Initialize()
InitializeComponent()
End Sub
Private Sub loadToolStripMenuItem_Click(sender As Object, e As EventArgs)
Dim openFileDialogLoad As New OpenFileDialog()
' Allow only PDF and PS files.
openFileDialogLoad.Filter = ImGearFileFormats.GetSavingFilter(ImGearSavingFormats.PDF) + "|" + ImGearFileFormats.GetSavingFilter(ImGearSavingFormats.PS)
If DialogResult.OK = openFileDialogLoad.ShowDialog() Then
Using inputStream As New FileStream(openFileDialogLoad.FileName, FileMode.Open, FileAccess.Read, FileShare.Read)
Try
' Load the entire the document.
igDocument = ImGearFileFormats.LoadDocument(inputStream)
Catch ex As ImGearException
' Perform error handling.
MessageBox.Show(ex.Message)
End Try
End Using
' Render first page.
renderPage(0)
End If
End Sub
Private Sub saveToolStripMenuItem_Click(sender As Object, e As EventArgs)
' Check if document exists.
If igDocument Is Nothing Then
Return
End If
Dim filename As String = ""
' Open File dialog. For this sample, just allow PDF or PS.
Dim savingFormat As ImGearSavingFormats = ImGearSavingFormats.PDF
Using fileDialogSave As New SaveFileDialog()
fileDialogSave.Filter = ImGearFileFormats.GetSavingFilter(ImGearSavingFormats.PDF) + "|" + ImGearFileFormats.GetSavingFilter(ImGearSavingFormats.PS)
fileDialogSave.OverwritePrompt = True
If fileDialogSave.ShowDialog(Me) <> DialogResult.OK Then
Return
End If
filename = fileDialogSave.FileName
End Using
' Save to output file.
Using outputStream As New FileStream(filename, FileMode.Create, FileAccess.ReadWrite)
Try
' Save the page in the requested format.
ImGearFileFormats.SaveDocument(igDocument, outputStream, 0, ImGearSavingModes.OVERWRITE, savingFormat, Nothing)
MessageBox.Show("Saved {0}", filename)
' Perform error handling.
Catch ex As Exception
MessageBox.Show(String.Format("Could not save file {0}. {1}", filename, ex.Message))
Return
End Try
End Using
End Sub
Private Sub renderPage(pageNumber As Integer)
' Create page to hold the content.
Dim igPage As ImGearPage = Nothing
' Load a single page from the loaded document.
Try
igPage = igDocument.Pages(pageNumber)
If igPage IsNot Nothing Then
' Create a new page display to prepare the page for being displayed.
Dim igPageDisplay As New ImGearPageDisplay(igPage)
' Associate the page display with the page view.
imGearPageView1.Display = igPageDisplay
' Cause the page view to repaint.
imGearPageView1.Invalidate()
currentPageIndex = pageNumber
toolStripStatusLabel1.Text = String.Format("{0} of {1}", pageNumber + 1, igDocument.Pages.Count)
End If
Catch ex As ImGearException
' Perform error handling.
MessageBox.Show(ex.Message)
End Try
End Sub
' Properly dispose of ImGearPDF and other objects.
Protected Overrides Sub OnFormClosed(e As FormClosedEventArgs)
ImGearPDF.Terminate()
imGearPageView1.Display = Nothing
End Sub
Private Sub nextPageToolStripMenuItem_Click(sender As Object, e As EventArgs)
If igDocument IsNot Nothing Then
If currentPageIndex < igDocument.Pages.Count - 1 Then
renderPage(currentPageIndex + 1)
End If
End If
End Sub
Private Sub previousPageToolStripMenuItem_Click(sender As Object, e As EventArgs)
If igDocument IsNot Nothing Then
If currentPageIndex > 0 Then
renderPage(currentPageIndex - 1)
End If
End If
End Sub
End Class
End Namespace |
- Now, let's go over some of the important areas in the sample code with more detail. There are 2 main data structures that are used in this sample code:
- The ImGearDocument that holds the entire loaded document
- The ImGearPage that holds a single page from that document (one page can only be displayed on the screen at a time).
- To initialize and support processing of PDF and PS files we need:
C# |
Copy Code |
// Initialize evaluation license.
ImGearEvaluationManager.Initialize();
ImGearEvaluationManager.Mode = ImGearEvaluationMode.Watermark;
// Add support for PDF and PS files.
ImGearCommonFormats.Initialize();
ImGearFileFormats.Filters.Insert(0, ImGearPDF.CreatePDFFormat());
ImGearFileFormats.Filters.Insert(0, ImGearPDF.CreatePSFormat());
ImGearPDF.Initialize(); |
VB.NET |
Copy Code |
' Initialize evaluation license.
ImGearEvaluationManager.Initialize()
ImGearEvaluationManager.Mode = ImGearEvaluationMode.Watermark
' Add support for PDF and PS files.
ImGearCommonFormats.Initialize()
ImGearFileFormats.Filters.Insert(0, ImGearPDF.CreatePDFFormat())
ImGearFileFormats.Filters.Insert(0, ImGearPDF.CreatePSFormat())
ImGearPDF.Initialize() |
- We load the document using:
C# |
Copy Code |
// Load the entire the document.
igDocument = ImGearFileFormats.LoadDocument(inputStream); |
VB.NET |
Copy Code |
' Load the entire the document.
igDocument = ImGearFileFormats.LoadDocument(inputStream) |
- Next, we will go over the code to render a page with the ImGearPageView control:
C# |
Copy Code |
private void renderPage(int pageNumber)
{
// Create page to hold the content.
ImGearPage igPage = null;
// Load a single page from the loaded document.
try
{
igPage = igDocument.Pages[pageNumber];
if (igPage != null)
{
// Create a new page display to prepare the page for being displayed.
ImGearPageDisplay igPageDisplay = new ImGearPageDisplay(igPage);
// Associate the page display with the page view.
imGearPageView1.Display = igPageDisplay;
// Cause the page view to repaint.
imGearPageView1.Invalidate();
currentPageIndex = pageNumber;
toolStripStatusLabel1.Text = string.Format("{0} of {1}", pageNumber + 1, igDocument.Pages.Count);
Application.DoEvents();
}
}
catch (ImGearException ex)
{
// Perform error handling.
MessageBox.Show(ex.Message);
}
} |
VB.NET |
Copy Code |
Private Sub renderPage(pageNumber As Integer)
' Create page to hold the content.
Dim igPage As ImGearPage = Nothing
' Load a single page from the loaded document.
Try
igPage = igDocument.Pages(pageNumber)
If igPage IsNot Nothing Then
' Create a new page display to prepare the page for being displayed.
Dim igPageDisplay As New ImGearPageDisplay(igPage)
' Associate the page display with the page view.
ImGearPageView1.Display = igPageDisplay
' Cause the page view to repaint.
ImGearPageView1.Invalidate()
currentPageIndex = pageNumber
ToolStripStatusLabel1.Text = String.Format("{0} of {1}", pageNumber + 1, igDocument.Pages.Count)
Application.DoEvents()
End If
Catch ex As ImGearException
' Perform error handling.
MessageBox.Show(ex.Message)
End Try
End Sub |
- To render one specific page we first need to create an ImGearPage object:
C# |
Copy Code |
// Create page to hold the content.
ImGearPage igPage = null; |
VB.NET |
Copy Code |
' Create empty IG document object.
Private igDocument As ImGearDocument = Nothing |
- You can access a page from a document using:
C# |
Copy Code |
igPage = igDocument.Pages[pageNumber]; |
VB.NET |
Copy Code |
igPage = igDocument.Pages(pageNumber) |
- To render a page, we need to first create an ImGearPageDisplay object that can be directly loaded into an ImGearPageView control:
C# |
Copy Code |
// Create a new page display to prepare the page for being displayed.
ImGearPageDisplay igPageDisplay = new ImGearPageDisplay(igPage); |
VB.NET |
Copy Code |
' Create a new page display to prepare the page for being displayed.
Dim igPageDisplay As New ImGearPageDisplay(igPage) |
- Last, but not least, we need to appropriately dispose of all used objects:
C# |
Copy Code |
// Properly dispose of ImGearPDF and other objects.
protected override void OnFormClosed(FormClosedEventArgs e)
{
ImGearPDF.Terminate();
imGearPageView1.Display = null;
} |
VB.NET |
Copy Code |
' Properly dispose of ImGearPDF and other objects.
Protected Overrides Sub OnFormClosed(e As FormClosedEventArgs)
ImGearPDF.Terminate()
ImGearPageView1.Display = Nothing
End Sub |
This sample project was created as a simple introduction to using the PDF functionality in ImageGear. For systems designed for production environments, you need to configure your projects more efficiently by only adding the resources that you need. For more information, refer to Deploying Your Product.