Introduction
This step-by-step tutorial will guide you through how to build a complete C# application that analyzes 1D barcodes using Visual Studio.
Steps
-
Create a new Console App (.NET Core) project:
-
Place the Project and Solution in the same folder to simplify the directory structure:
-
Add the Barcode Xpress SDK to your project:
To let Visual Studio manage your Barcode Xpress dll, add the NuGet package to your project by browsing:
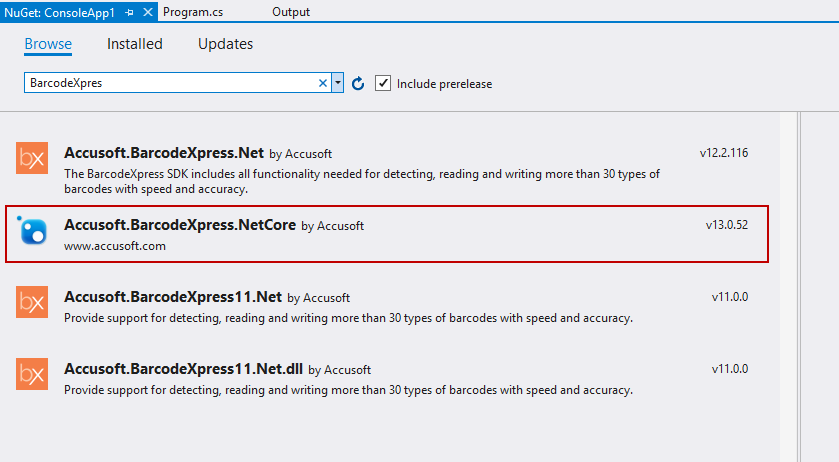If you already have the Barcode Xpress SDK installed on your computer, you can instead add a reference to your project to use the local version:
-
Add using statements to your generated Program.cs:
using System; using Accusoft.BarcodeXpressSdk; namespace MyProject {
-
Create instance of Accusoft.BarcodeXpressSdk.BarcodeXpress and open an image stream:
using System; using System.Drawing; using Accusoft.BarcodeXpressSdk; namespace MyProject { class Program { static void Main(string[] args) { BarcodeXpress barcodeXpress = new BarcodeXpress("."); using (Stream stream = File.OpenRead("barcode.bmp")) { } } } }
-
Pass the Stream to BarcodeXpress using the Analyze(Stream) method and print out barcode results.
namespace MyProject { class Program { static void Main(string[] args) { BarcodeXpress barcodeXpress = new BarcodeXpress("."); using (Stream stream = File.OpenRead("barcode.bmp")) { Accusoft.BarcodeXpressSdk.Result[] results = barcodeXpress.reader.Analyze(stream); if (results.Length > 0) { foreach (Accusoft.BarcodeXpressSdk.Result result in results) { Console.WriteLine("{0} : {1}", result.BarcodeType.ToString(), result.BarcodeValue); } } else { Console.WriteLine("No Barcodes Found."); } } } } }
BarcodeXpress.reader.Analyze returns an array of Result objects which contain information about all barcodes detected on the page. You can iterate over that array to access all known properties of each barcode.
-
Finally, clean up the Barcode Xpress object by releasing unmanaged resources using the Dispose() method:
namespace MyProject { class Program { static void Main(string[] args) { BarcodeXpress barcodeXpress = new BarcodeXpress("."); using (Stream stream = File.OpenRead("barcode.bmp")) { Accusoft.BarcodeXpressSdk.Result[] results = barcodeXpress.reader.Analyze(stream); if (results.Length > 0) { foreach (Accusoft.BarcodeXpressSdk.Result result in results) { Console.WriteLine("{0} : {1}", result.BarcodeType.ToString(), result.BarcodeValue); } } else { Console.WriteLine("No Barcodes Found."); } } barcodeXpress.Dispose(); } } }