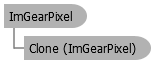
'Declaration <DefaultMemberAttribute("Item")> Public NotInheritable Class ImGearPixel
'Usage Dim instance As ImGearPixel
[DefaultMember("Item")] public sealed class ImGearPixel
[DefaultMember("Item")] public __gc __sealed class ImGearPixel
[DefaultMember("Item")] public ref class ImGearPixel sealed
For simplicity, ImGearPixel does not contain any information about channels depths and color space. Therefore pixels that are identical in structure (ImGearPixel
constructor parameters) may belong to images with significantly different channels, structure and color space.
NOTE: for initialization of pixel value for using it with bitonal images, i.e. Run-Ends DIBs, please use 0 value for black color, and 1 value for white color.
// Create a pixel for an image with an 8bit palette. ImGearPixel igPixelIndexed = new ImGearPixel(1, 8); // Assign the pixel palette position 176. igPixelIndexed[0] = 176; // Create a 512x512 8bit indexed image. ImGearPage igPageIndex = new ImGearRasterPage(512, 512, new ImGearColorSpace(ImGearColorSpaceIDs.I), new int[] { 8 }, true); // Allocate a new palette igPageIndex.DIB.Palette = new ImGearRGBQuad[256]; // Set the 176th palette entry to green. igPageIndex.DIB.Palette[176].Red = 0; igPageIndex.DIB.Palette[176].Green = 127; igPageIndex.DIB.Palette[176].Blue = 0; // Update the full image with this pixel. for (int height = 0; height < igPageIndex.DIB.Height; height++) for (int width = 0; width < igPageIndex.DIB.Width; width++) igPageIndex.DIB.UpdatePixelFrom(width, height, igPixelIndexed); // Create a pixel for a 32bit CMYK image. ImGearPixel igPixelCMYK = new ImGearPixel(4, 8); // Assign the pixel a blue color. igPixelCMYK[0] = 255; igPixelCMYK[1] = 255; igPixelCMYK[2] = 0; igPixelCMYK[3] = 127; // Creates a 512x512 32bit CMYK image. ImGearPage igPageCMYK = new ImGearRasterPage(512, 512, new ImGearColorSpace(ImGearColorSpaceIDs.CMYK), new int[] { 8, 8, 8, 8 }, true); for (int height = 0; height < igPageCMYK.DIB.Height; height++) for (int width = 0; width < igPageCMYK.DIB.Width; width++) igPageCMYK.DIB.UpdatePixelFrom(width, height, igPixelCMYK);
'Create a pixel for an image with an 8bit palette. Dim igPixelIndexed As ImGearPixel = New ImGearPixel(1, 8) 'Assign the pixel palette position 176. igPixelIndexed(0) = 176 'Create a 512x512 8bit indexed image. Dim igPageIndex As ImGearPage = New ImGearRasterPage(512, 512, _ New ImGearColorSpace(ImGearColorSpaceIDs.I), _ New Integer() {8}, True) 'Allocate a new palette ReDim igPageIndex.DIB.Palette(256) 'Set the 176th palette entry to green. igPageIndex.DIB.Palette(176).Red = 0 igPageIndex.DIB.Palette(176).Green = 127 igPageIndex.DIB.Palette(176).Blue = 0 'Update the full image with this pixel. For height As Integer = 0 To igPageIndex.DIB.Height - 1 For width As Integer = 0 To igPageIndex.DIB.Width - 1 igPageIndex.DIB.UpdatePixelFrom(width, height, igPixelIndexed) Next Next 'Create a pixel for a 32bit CMYK image. Dim igPixelCMYK As ImGearPixel = New ImGearPixel(4, 8) 'Assign the pixel a blue color. igPixelCMYK(0) = 255 igPixelCMYK(1) = 255 igPixelCMYK(2) = 0 igPixelCMYK(3) = 127 'Creates a 512x512 32bit CMYK image. Dim igPageCMYK As ImGearPage = New ImGearRasterPage(512, 512, _ New ImGearColorSpace(ImGearColorSpaceIDs.CMYK), _ New Integer() {8, 8, 8, 8}, True) For height As Integer = 0 To igPageCMYK.DIB.Height - 1 For width As Integer = 0 To igPageCMYK.DIB.Width - 1 igPageCMYK.DIB.UpdatePixelFrom(width, height, igPixelCMYK) Next Next
System.Object
ImageGear.Core.ImGearPixel